スタンダード
ノーマルヘッダー
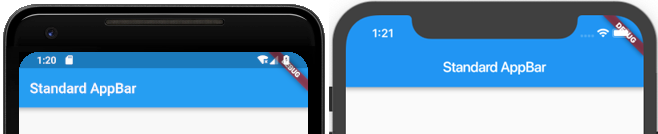
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Standard AppBar',
home: Scaffold(
appBar:AppBar(
title: Text('Standard AppBar'),
)
)
);
}
}
アイコン

class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar:AppBar(
title: const Text('Standard AppBar'),
actions: <Widget>[
IconButton(
icon: Icon(Icons.settings),
onPressed: () {
// Pressed Action
},
),
IconButton(
icon: Icon(Icons.menu),
onPressed: () {
// Pressed Action
},
),
],
)
)
);
}
}
ポップアップメニュー
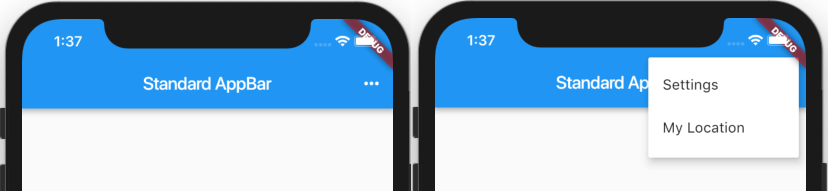
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar:AppBar(
title: const Text('Standard AppBar'),
actions: <Widget>[
// overflow menu
PopupMenuButton<Choice>(
onSelected: (Choice choice) {
// Selected Action
},
itemBuilder: (BuildContext context) {
return choices.map((Choice choice) {
return PopupMenuItem<Choice>(
value: choice,
child: Text(choice.title),
);
}).toList();
},
),
],
)
)
);
}
}
class Choice {
const Choice({this.title, this.icon});
final String title;
final IconData icon;
}
const List<Choice> choices = const <Choice>[
const Choice(title: 'Settings', icon: Icons.settings),
const Choice(title: 'My Location', icon: Icons.my_location),
];
センターロゴヘッダー
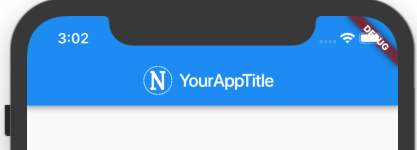
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Standard AppBar',
home: Scaffold(
appBar:AppBar(
title: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Image.asset(
'assets/logo.png',
fit: BoxFit.contain,
height: 32,
),
Container(
padding: const EdgeInsets.all(8.0),
child: Text('YourAppTitle')
)
],
),
)
)
);
}
}
※イメージの読み込み設定をしていない場合は
こちら左寄せロゴヘッダー
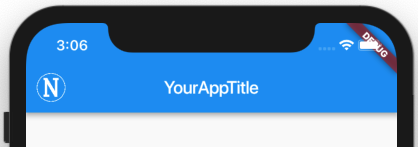
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Standard AppBar',
home: Scaffold(
appBar:AppBar(
centerTitle: true,
title: const Text('YourAppTitle'),
leading: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Image.asset(
'assets/logo.png',
fit: BoxFit.contain,
height: 32,
),
]
),
)
)
);
}
}
※イメージの読み込み設定をしていない場合は
こちら